Q. If sum of two integer is 14 and if one of them is 8 then find other integer. Ans: Well, this is very simple. Simply, one integer is 8 and sum of two integers is 14. So, we need to subtract the number 8 from their sum of 14 to get the second number. So, the second number is: 14 - 8 = 6 C-Program of this task Code---> #include<stdio.h> int main () { int num1 = 8 , num2 , sum = 14 ; num2 = sum - num1 ; printf ( "The second number is: %d" , num2 ); return 0 ; }
In this C program we will multiply two matrices and print the result on the screen.The elements of the matrices will be taken from the user.
input:
The elements of matrices.output:
The Multiplication of the two matrices.
CODE---->
#include <stdio.h>
void input(int first[10][10],int second[10][10],int r1,int c1,int r2,int c2)
{
int i,j;
printf("\nEnter elements of matrix 1:\n");
for (i=0;i<r1;i++)
{
for (j=0;j<c1;j++)
{
printf("Enter data: ");
scanf("%d",&first[i][j]);
}
}
printf("\nEnter elements of matrix 2:\n");
for(i=0;i<r2;i++)
{
for(j=0;j<c2;j++)
{
printf("Enter data: ");
scanf("%d",&second[i][j]);
}
}
}
void multiply(int first[10][10],int second[10][10],int mul[10][10],int r1,int c1,int r2,int c2)
{
int i,j,k;
// Initializing elements of matrix mul to 0.
for(i=0;i<r1;i++)
{
for(j=0;j<c2;j++)
{
mul[i][j]=0;
}
}
// Multiplying first and second matrices and storing in mul.
for(i=0;i<r1;i++)
{
for(j=0;j<c2;j++)
{
for(k=0;k<c1;k++)
{
mul[i][j]=mul[i][j]+first[i][k]*second[k][j];
}
}
}
}
void display(int mul[10][10],int r1,int c2)
{
int i,j;
printf("\nOutput Matrix:\n");
for(i=0;i<r1;i++)
{
for(j=0;j<c2;j++)
{
printf("%d ",mul[i][j]);
if(j==c2-1)
printf("\n");
}
}
}
int main()
{
int first[10][10],second[10][10],mul[10][10],r1,c1,r2,c2;
printf("Enter No. of rows and column for the first matrix: ");
scanf("%d%d",&r1,&c1);
printf("Enter No. of rows and column for the second matrix: ");
scanf("%d%d",&r2,&c2);
// Taking input until columns of the first matrix is equal to the rows of the second matrix
while(c1!=r2)
{
printf("\nError! Enter rows and columns again.\n");
printf("Enter rows and columns for the first matrix: ");
scanf("%d%d",&r1,&c1);
printf("Enter rows and columns for the second matrix: ");
scanf("%d%d",&r2,&c2);
}
// Function to take matrices data
input(first,second,r1,c1,r2,c2);
// Function to multiply two matrices.
multiply(first,second,mul,r1,c1,r2,c2);
// Function to display resultant matrix after multiplication.
display(mul,r1,c2);
return 0;
}
Download the C-Program file of this Program.
RESULT :
Enter No. of rows and column for the first matrix: 2 2 Enter No. of rows and column for the second matrix: 2 2 Enter elements of matrix 1: Enter data: 2 Enter data: 1 Enter data: 3 Enter data: 4 Enter elements of matrix 2: Enter data: 1 Enter data: -2 Enter data: -1 Enter data: 1 Output Matrix: 1 -3 -1 -2 -------------------------------- Process exited after 18.96 seconds with return value 0 Press any key to continue . . .
Images for better understanding :
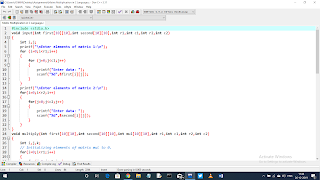
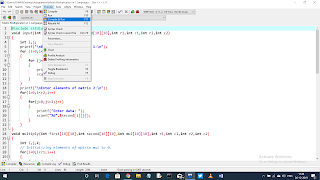
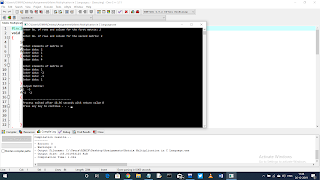
It gives me an immense pleasure to go through your posts from time to time.
ReplyDeleteBecause of their unique content and presentation. I wish you a success and hope you
keep writing more and more such posts.
Read my blog: Regression Testing: Ensuring Code Stability Across Versions