Q. If sum of two integer is 14 and if one of them is 8 then find other integer. Ans: Well, this is very simple. Simply, one integer is 8 and sum of two integers is 14. So, we need to subtract the number 8 from their sum of 14 to get the second number. So, the second number is: 14 - 8 = 6 C-Program of this task Code---> #include<stdio.h> int main () { int num1 = 8 , num2 , sum = 14 ; num2 = sum - num1 ; printf ( "The second number is: %d" , num2 ); return 0 ; }
In this C program we will concatenate two strings without using strcat()fuction. The Strings will be given by the User.
If gets() function doesn't working for you, change the gets() lines by
scanf("%[^\n]%*c", str1);
and scanf("%[^\n]%*c", str2);
Learn about different string functions and their use: Different String Functions
input:
The Strings.output:
The concatenated string will be printed on the screen. (i.e: First string + Second string)
CODE---->
#include<stdio.h>
#include<stdlib.h>
void STRCAT(char *s1,char *s2)
{
while(*s1!='\0')
{
s1++;
}
while(*s2!='\0')
{
*s1=*s2;
s1++;
s2++;
}
*s1='\0';
}
int main()
{
char str1[100],str2[100];
printf("Please, Enter the First String: ");
gets(str1);
printf("Please, Enter the second String: ");
gets(str2);
STRCAT(str1,str2);
printf("After concatenation: %s",str1);
return 0;
}
Download the C-Program file of this Program.
RESULT :
Please, Enter the First String: Programming is fun Please, Enter the second String: with ProgramJoy After concatenation: Programming is fun with ProgramJoy -------------------------------- Process exited after 35.27 seconds with return value 0 Press any key to continue . . .
Images for better understanding :
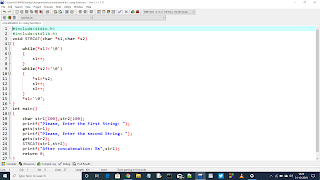
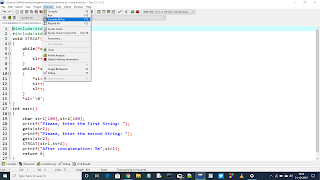
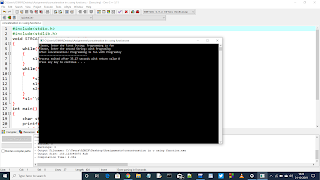
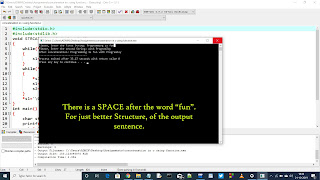
Comments
Post a Comment