Q. If sum of two integer is 14 and if one of them is 8 then find other integer. Ans: Well, this is very simple. Simply, one integer is 8 and sum of two integers is 14. So, we need to subtract the number 8 from their sum of 14 to get the second number. So, the second number is: 14 - 8 = 6 C-Program of this task Code---> #include<stdio.h> int main () { int num1 = 8 , num2 , sum = 14 ; num2 = sum - num1 ; printf ( "The second number is: %d" , num2 ); return 0 ; }
In this C++ program we will add and multiply two matrices and print the result on the screen.The elements of the matrices will be taken from the user.
input:
The elements of matrices.output:
The addition and multiplication of the two matrices.
CODE---->
#include<iostream>
using namespace std;
class matrix{
private:
int mat[10][10];
public:
matrix()
{
int i,j;
for(i=1;i<=3;i++)
{
for(j=1;j<=3;j++)
{
mat[i][j]=0;
}
}
}
int getdata()
{
int i,j,n;
for(i=1;i<=3;i++)
{
for(j=1;j<=3;j++)
{
cout<< "\nEnter data(" <<i <<"," <<j << "): ";
cin>> n;
mat[i][j]=n;
}
}
}
int displaydata()
{
int i,j;
cout<< "\n";
for(i=1;i<=3;i++)
{
for(j=1;j<=3;j++)
{
cout<< mat[i][j] <<"\t";
}
cout<<"\n";
}
}
matrix operator+(matrix ob2)
{
int i,j;
int x[10][10];
for(i=1;i<=3;i++)
{
for(j=1;j<=3;j++)
{
x[i][j]=0;
x[i][j]=mat[i][j]+ob2.mat[i][j];
}
}
cout<< "\nThe addition result is: \n";
cout<< "---------------------------\n";
for(i=1;i<=3;i++)
{
for(j=1;j<=3;j++)
{
cout<< x[i][j] <<"\t";
}
cout<<"\n";
}
}
matrix operator*(matrix ob2)
{
int i,j,k;
int x[20][20];
for(i=1;i<=3;i++)
{
for(j=1;j<=3;j++)
{
x[i][j]=0;
for(k=1;k<=3;k++)
{
x[i][j]+=mat[i][k]*ob2.mat[k][j];
}
}
}
cout<< "\nThe multiplication result is: \n";
cout<< "---------------------------------\n";
for(i=1;i<=3;i++)
{
for(j=1;j<=3;j++)
{
cout<< x[i][j] <<"\t";
}
cout<<"\n";
}
}
};
int main()
{
matrix ob1,ob2,ob3,ob4;
cout<< "Enter data for first matrix: ";
ob1.getdata();
cout<< "\nFirst created matrix is: \n";
cout<< "-------------------------";
ob1.displaydata();
cout<< "\nEnter data for second matrix: ";
ob2.getdata();
cout<< "\nSecond created matrix is: \n";
cout<< "--------------------------";
ob2.displaydata();
ob3=ob1+ob2;
ob4=ob1*ob2;
return 0;
}
Download the C++ file of this Program.
RESULT :
Enter data for first matrix: Enter data(1,1): -1 Enter data(1,2): 5 Enter data(1,3): 8 Enter data(2,1): -4 Enter data(2,2): 0 Enter data(2,3): 12 Enter data(3,1): 19 Enter data(3,2): -8 Enter data(3,3): 9 First created matrix is: ------------------------- -1 5 8 -4 0 12 19 -8 9 Enter data for second matrix: Enter data(1,1): 3 Enter data(1,2): -2 Enter data(1,3): 0 Enter data(2,1): 7 Enter data(2,2): 15 Enter data(2,3): 6 Enter data(3,1): -10 Enter data(3,2): 18 Enter data(3,3): 19 Second created matrix is: -------------------------- 3 -2 0 7 15 6 -10 18 19 The addition result is: --------------------------- 2 3 8 3 15 18 9 10 28 The multiplication result is: --------------------------------- -48 221 182 -132 224 228 -89 4 123 -------------------------------- Process exited after 71.35 seconds with return value 0 Press any key to continue . . .
Images for better understanding :
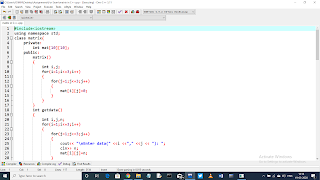
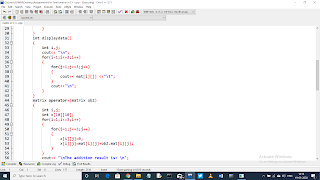
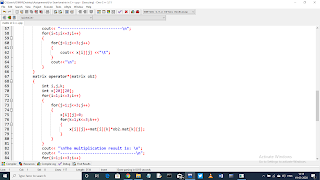
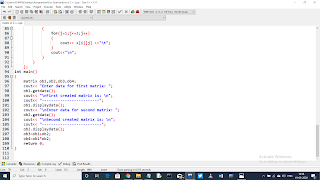
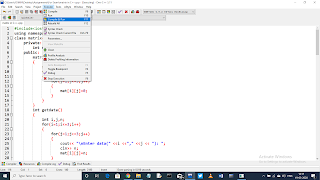
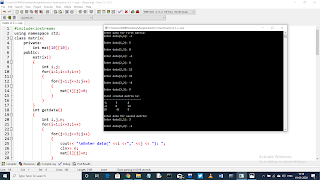
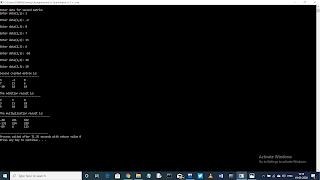
Comments
Post a Comment