Q. If sum of two integer is 14 and if one of them is 8 then find other integer. Ans: Well, this is very simple. Simply, one integer is 8 and sum of two integers is 14. So, we need to subtract the number 8 from their sum of 14 to get the second number. So, the second number is: 14 - 8 = 6 C-Program of this task Code---> #include<stdio.h> int main () { int num1 = 8 , num2 , sum = 14 ; num2 = sum - num1 ; printf ( "The second number is: %d" , num2 ); return 0 ; }
In this C program we will calculate the Factorial of a number(integer) using RECURSION method and print the result, in the screen. The number(integer) will be taken from the user.
input:
The number(integer) (i.e. 5,7 etc.)output:
The Factorial of the number will be printed on the screen.
CODE---->
#include<stdio.h>
#include<conio.h>
int fact(int x); //Function declaration
main()
{
int x,ans;
printf(" Enter the number : ");
scanf("%d",&x);
ans=fact(x); //Function Call
printf("\n The factorial of %d is : %d",x,ans);
getch();
} //Main function ends here
int fact(int x) //Function Structure
{
int f;
if(x==0)
return 1;
else
f=x*fact(x-1);
return f;
}
Caution :
In this C-program we have used an integer number as an input and the variable where the calculated result is stored, is also an integer. The range of an integer variable is between -32,768 to 32,767, so, the calculation of a factorial must be in this range. otherwise, it will show you a garbage value or wrong calculation. You can prevent this problem by using "Long long signed integer type(%lli)". Capable of containing at least the [−9,223,372,036,854,775,807, +9,223,372,036,854,775,807] range.
But, this C-program is not a wrong Program.It's just about range.
RESULT:
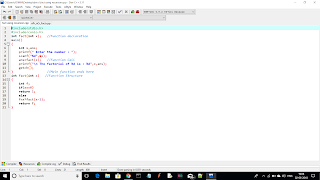
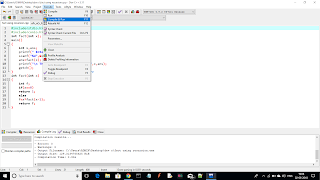
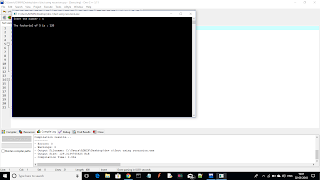
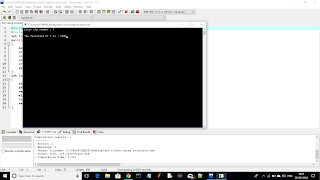
Comments
Post a Comment