Q. If sum of two integer is 14 and if one of them is 8 then find other integer. Ans: Well, this is very simple. Simply, one integer is 8 and sum of two integers is 14. So, we need to subtract the number 8 from their sum of 14 to get the second number. So, the second number is: 14 - 8 = 6 C-Program of this task Code---> #include<stdio.h> int main () { int num1 = 8 , num2 , sum = 14 ; num2 = sum - num1 ; printf ( "The second number is: %d" , num2 ); return 0 ; }
In this C-program we will Convert the Octal Number into Decimal number and Decimal Number into Octal Number. The number will be taken from the user.
input:
The number.(i.e : 5,6,9[Decimal Number] or 12,57,567[Octal Number] etc.)output:
Octal number will be converted into Decimal number OR Decimal number will be converted into Octal number.
CODE---->
#include<stdio.h>
#include<conio.h>
#include<math.h>
main()
{
int oct_num,oct,dec_num,dec,remainder,i,n;
printf("***************Welcome to Converter***************");
printf("\n\n\n1.Octal to Decimal\n\n2.Decimal to Octal\n\n Enter Your choice : ");
scanf("%d",&n);
switch(n)
{
case 1:
printf("\nPlease, Enter the Octal number : ");
scanf("%d",&oct_num);
oct=oct_num;
i=0;
dec=0;
while(oct_num!=0)
{
remainder=oct_num%10;
dec=dec+remainder*pow(8,i);
i++;
oct_num=oct_num/10;
}
printf("\nThe decimal of %d is : %d",oct,dec);
break;
case 2:
printf("\nPlease, Enter the Decimal number : ");
scanf("%d",&dec_num);
dec=dec_num;
i=1;
oct=0;
while(dec_num!=0)
{
remainder=dec_num%8;
dec_num=dec_num/8;
oct=oct+remainder*i;
i=i*10;
}
printf("\nThe octal of %d is : %d",dec,oct);
break;
default:
printf("\n SORRY !!! You have Entered a wrong choice.");
break;
}
getch();
}
RESULT:
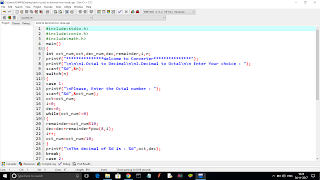
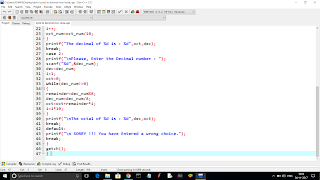
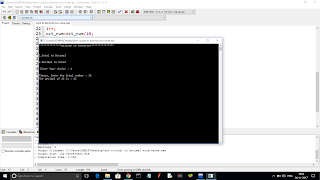
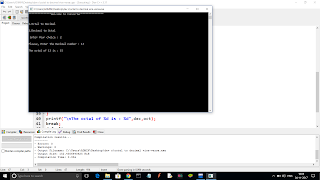
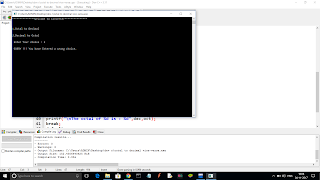
This comment has been removed by the author.
ReplyDeleteThis comment has been removed by the author.
ReplyDelete